What is a MVW framework?
The abbreviation stands for 'Model - View - Whatever'. Well there are many different JavaScript frameworks available , all invented for the same purpose. They all try to separate the
presentation logic from the business logic where JavaScript holds the
model and logic, and html the presentation layer.
Quick overview :
- You can change the model without changing the view and vice-versa
- Unit testing is easy
For several years +AngularJS was closer to MVC (or rather one of its client-side variants), but over time and thanks to many refactorings and api improvements, it's now closer to MVVM – the $scope object could be considered the ViewModel that is being decorated by a function that we call a Controller.
Being able to categorize a framework and put it into one of the MV* buckets has some advantages. It can help developers get more comfortable with its apis by making it easier to create a mental model that represents the application that is being built with the framework. It can also help to establish terminology that is used by developers.
Having said, I'd rather see developers build kick-ass apps that are well-designed and follow separation of concerns, than see them waste time arguing about MV* nonsense. And for this reason, I hereby declare AngularJS to be MVW framework - Model-View-Whatever. Where Whatever stands for "whatever works for you".
Angular gives you a lot of flexibility to nicely separate presentation logic from business logic and presentation state. Please use it fuel your productivity and application maintainability rather than heated discussions about things that at the end of the day don't matter that much.
Why to use JavaScript ?
Nowadays all web pages extensively use JavaScript which cause several advantages:
- More interactive and responsive UI
- Save bandwidth
- Save CPU consumption on the web server
Components in AngularJS apps:
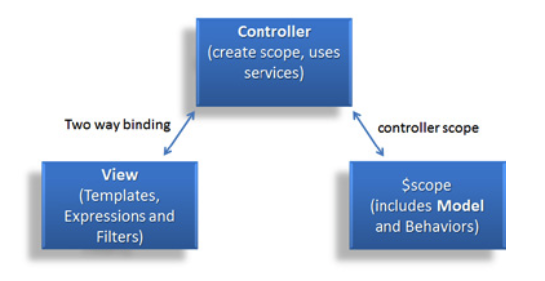
Controller :
- Controller should be just an interlayer between model and view. Try to make it as thin as possible.
- It is highly recommended to avoid business logic in controller. It should be moved to model.
- Controller may communicate with other controllers using method invocation (possible when children wants to communicate with parent) or $emit, $broadcast and $on methods. The emitted and broadcasted messages should be kept to a minimum.
- Controller should not care about presentation or DOM manipulation.
- Try to avoid nested controllers. In this case parent controller is interpreted as model. Inject models as shared services instead.
- Scope in controller should be used for binding model with view and
encapsulating View Model as for Presentation Model design pattern.
Scope :
When doing bidirectional binding (ng-model) make sure you don't bind directly to the scope properties.
Model :
Model provides an excellent way to separate data and display.
Models are prime candidates for unit testing, as they typically have exactly one dependency (some form of event emitter, in common case the $rootScope) and contain highly testable domain logic.
- Model should be considered as an implementation of particular unit. It is based on single-responsibility-principle. Unit is an instance that is responsible for its own scope of related logic that may represent single entity in real world and describe it in programming world in terms of data and state.
- Model should encapsulate your application’s data and provide an API to access and manipulate that data.
- Model should be portable so it can be easily transported to similar application.
- By isolating unit logic in your model you have made it easier to locate, update, and maintain.
- Model can use methods of more general global models that are common for the whole application.
- Try to avoid composition of other models into your model using dependency injection if it is not really dependent to decrease components coupling and increase unit testability and usability.
- Try to avoid using event listeners in models. It makes them harder to test and generally kills models in terms of single-responsibility-principle.
View :
Services :
Data Binding :
Directives :
Filters :
Validation :
Testable :
Nice article........................
ReplyDelete